Coding Best Practices — Write Clean Code
Clean code is the foundation of a robust, maintainable software project. It’s code that’s easy to understand, modify, and extend. It’s not just about making it work; it’s about making it work well, both now and in the future.
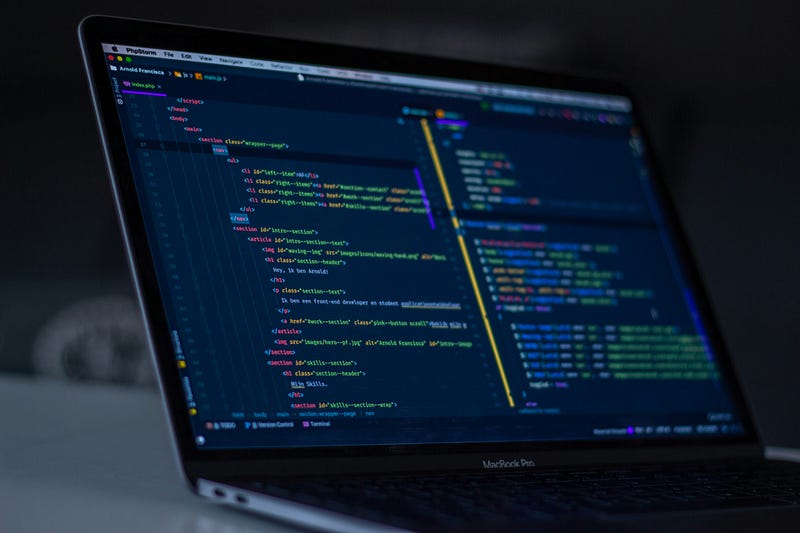
Core Principles for Cleaner Code
- Meaningful Names: Choose descriptive names for variables, functions, and classes. This makes your code self-documenting. Avoid abbreviations and single-letter variables.
// Good
int customerAge;
function calculateTotalPrice()
// Avoid
int x;
function calcTot()
- Functions Should Do One Thing: Each function should have a single responsibility. This keeps them short, focused, and easy to test.
// Good
function validateEmail(email)
function sendWelcomeEmail(email)
// Avoid
function registerUserAndSendEmail(email)
- Keep It Short: Limit functions to 8 lines or less, when possible. This improves readability and prevents overly complex logic.
// Good
function calculateAverage(numbers) {
let sum = 0;
for (let num of numbers) {
sum += num;
}
return sum / numbers.length;
}
- Comments Are for Clarification: Write clear, concise comments to explain the “why” behind your code, not the “what.”
// Good
// This function calculates the average to avoid division by zero.
function calculateSafeAverage(numbers) { ... }
// Avoid
// Loop through numbers and add them up
function calculateAverage(numbers) { ... }
- Limit Class Size: Keep classes to 100 lines or less. Larger classes become harder to manage. Break them down into smaller, more cohesive units.
- Consistent Formatting: Follow a consistent style guide for indentation, spacing, and naming conventions. This makes your code visually consistent and easier to scan.
Additional Tips
- DRY (Don’t Repeat Yourself): Refactor repeated code into reusable functions or modules.
- Error Handling: Implement robust error handling to prevent crashes and unexpected behavior.
- Testing: Write unit tests to verify your code’s correctness and catch regressions.
- Refactoring: Regularly revisit and improve your code to keep it clean and maintainable.
Example: Clean vs. Less Clean Code
// Less Clean
function c(a,b){
var x=a+b;
if(x>10){
return x*2;
}else{
return x/2;
}
}
// Cleaner
function calculateResult(input1, input2) {
const sum = input1 + input2;
return (sum > 10) ? sum * 2 : sum / 2;
}